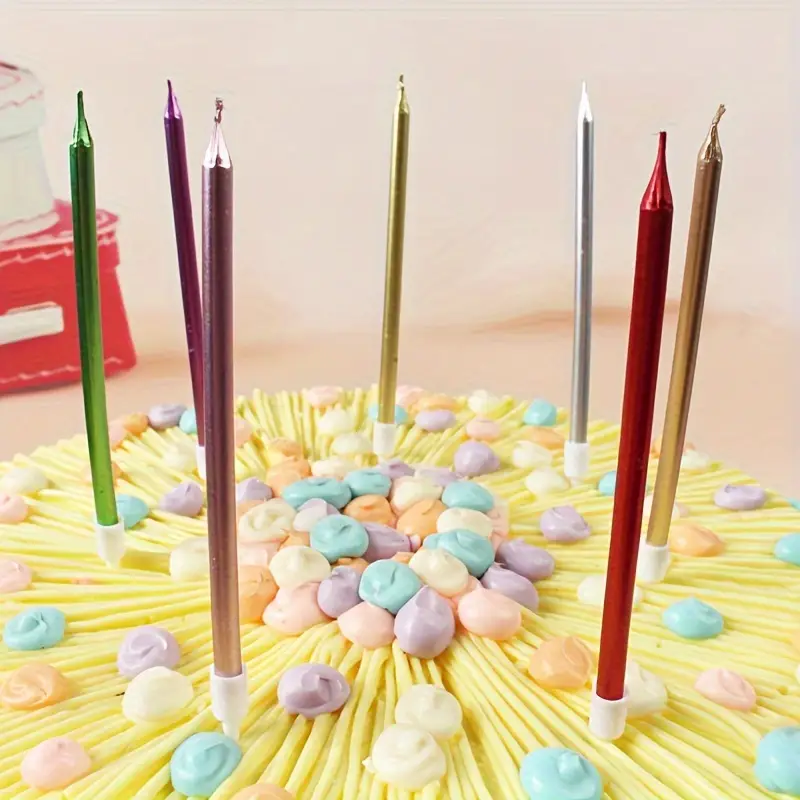
Candele A Matita Da 5 Pezzi, Candele Di Compleanno Con Vernice Per Feste Creative Per La Cottura Di Torte, Candele Di Compleanno, Candele Per Festival, Decorazioni Per La Casa, Decorazioni Per La
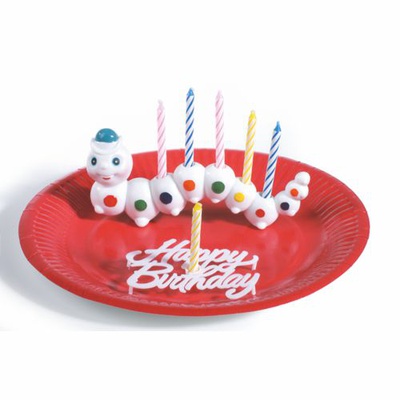
Supporti per candeline da compleanno 6,5 cm x 15 cm ''Worm'' con 6 candele | Pierrot srl - Dal 1983 Professionali Emozioni

Candele di compleanno extra lunghe per torta in colore pastello, confezione da 12 pezzi. : Amazon.it: Casa e cucina

Numero candele di compleanno 1 2 3 4 5 6 7 8 9 0 nastro candele di compleanno per bambini per torta forniture per feste numero candele decorazione - AliExpress

Candele Compleanno, 12 PCS Candele di Compleanno Metalliche Candeline Lunghe Compleanno Sottili con Supporti per Anniversario, Partylite Candele Decorazioni Torte Compleanno (Champagne) : Amazon.it: Casa e cucina

1pc candele romantico buon compleanno arte musicale girevole fiore di loto candela torta fai da te candela decorazione per regali di compleanno per bambini - AliExpress

Relaxdays Candeline per Compleanno, Set da 152 Candele e Supporti, per Torte e Party, Oggetti Decorativi, 6 cm, Multicolore, Set da 1 : Amazon.it: Casa e cucina
