
Chanel Allure Homme Sport Cologne Eau de Cologne (uomo) - tester 100 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.

Chanel Allure Homme Eau de Toilette (uomo) - tester 50 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.

Perfume Tester Chanel Allure Homme Sport, Beauty & Personal Care, Fragrance & Deodorants on Carousell
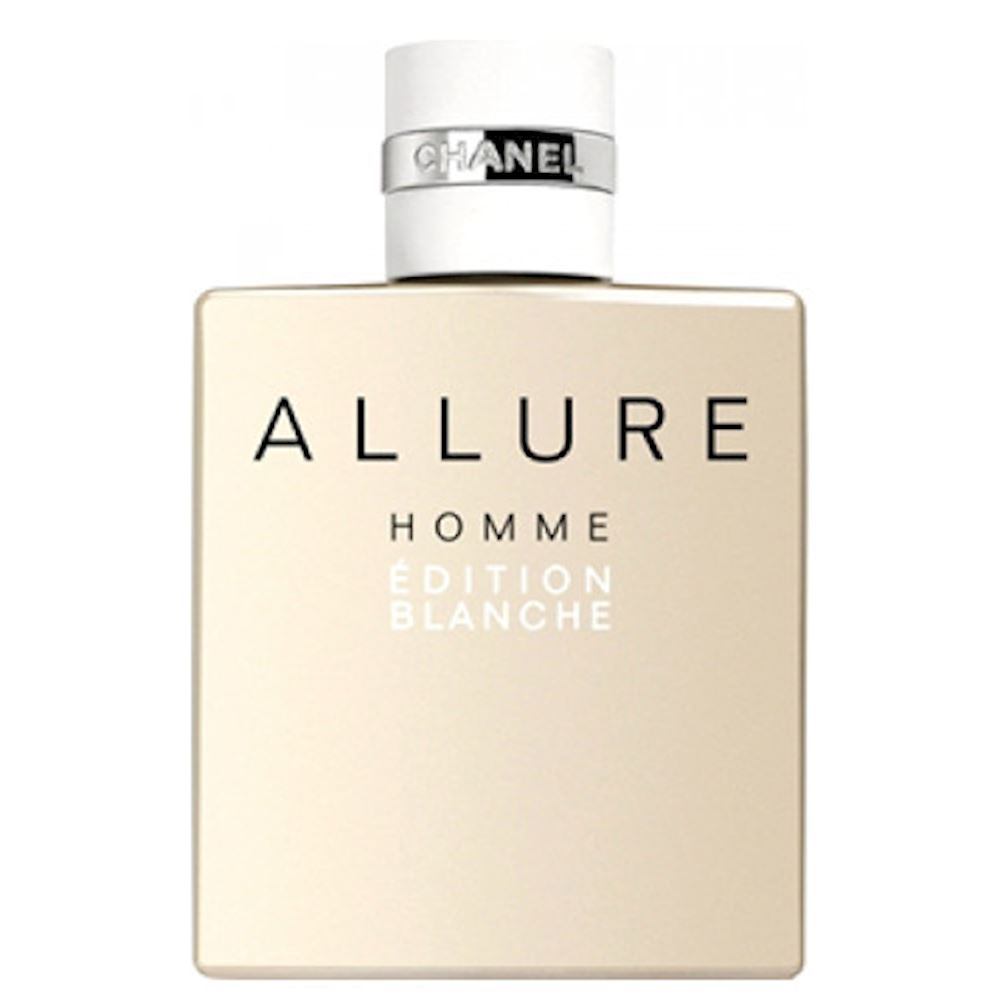