
Unguento curativo per neonati da 59ml. Crema curativa naturale certificata per eczema neonati, crosta lattea (dermatite seborroica infantile), naso screpolato, eruzioni cutanee, orticaria e altro : Amazon.it: Prima infanzia

Unguento curativo per neonati da 59ml. Crema curativa naturale certificata per eczema neonati, crosta lattea (dermatite seborroica infantile), naso screpolato, eruzioni cutanee, orticaria e altro : Amazon.it: Prima infanzia
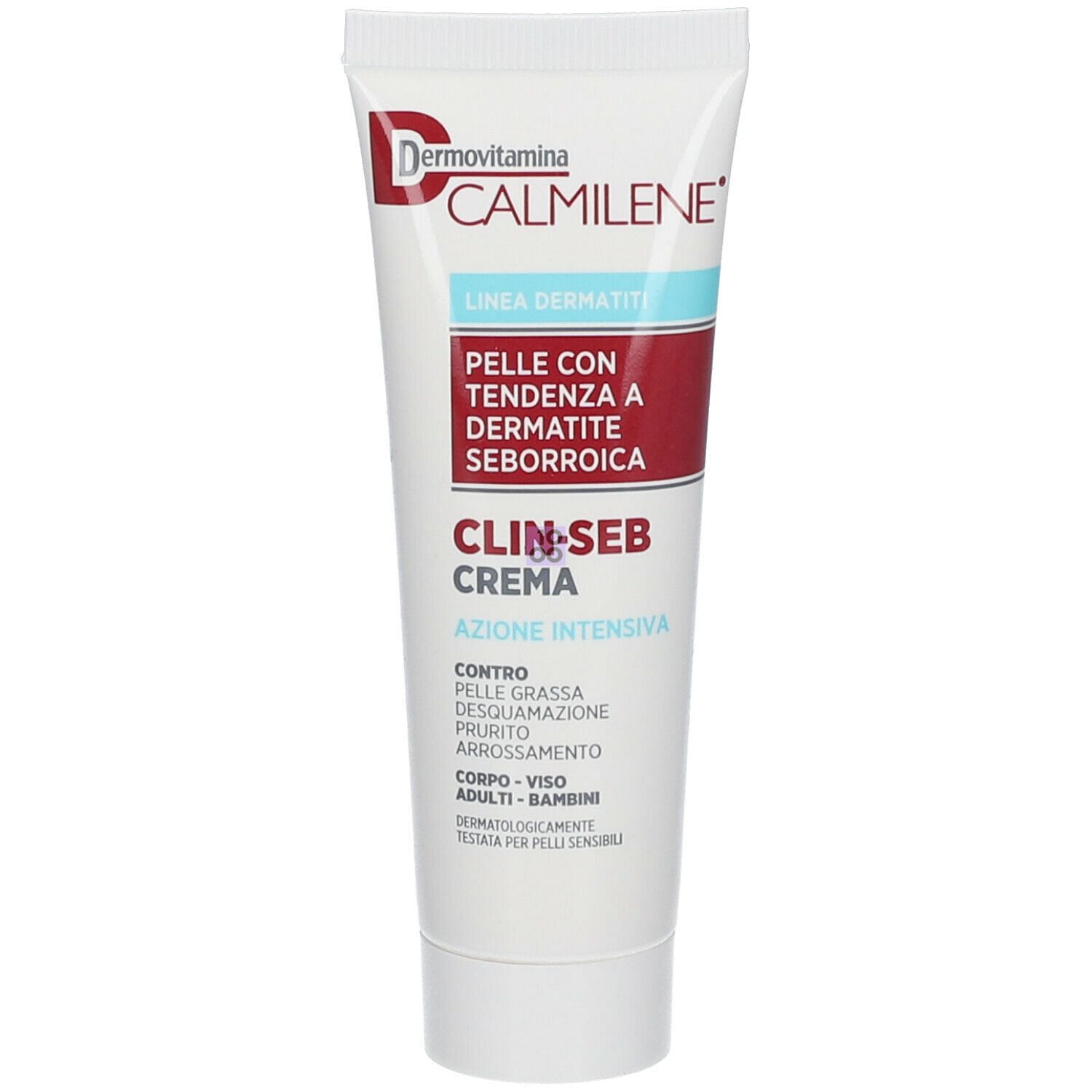
Dermovitamina Calmilene Clin Seb Crema Azione Intensiva Per Pelle Con Tendenza A Dermatite Seborroica 50 Ml | 1000farmacie
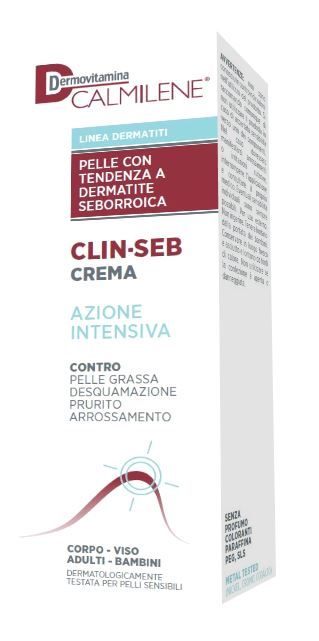
Dermovitamina calmilene clin-seb crema azione intensiva per pelle con tendenza a dermatite seborroica 50 ml | Farmacia Online
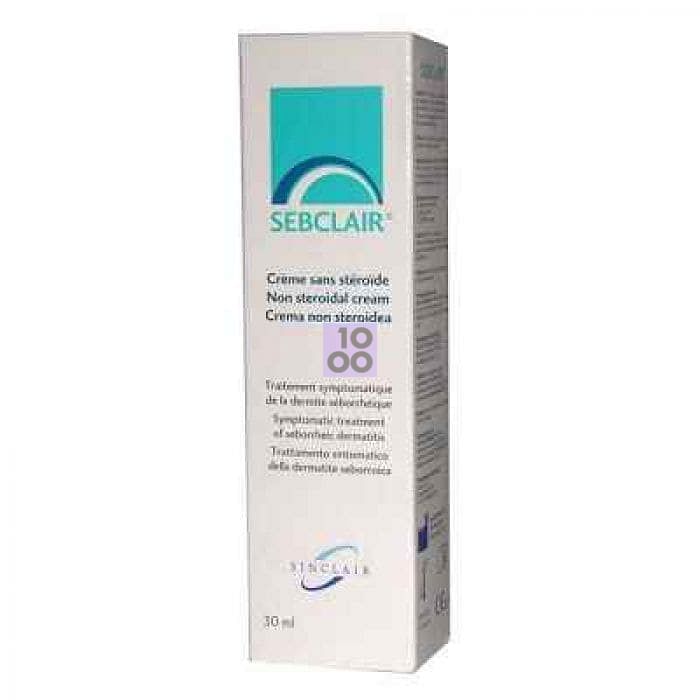