
kit controllo del peso, frullato formula 1 550g sostituto del pasto + 14 barrette proteiche gusto agrumi, scegli il tuo gusto preferito (Caffellatte) : Amazon.it: Salute e cura della persona
Herbalife Nutrition - Rimani motivato e non cedere alle cattive tentazioni! Le Barrette proteiche di Herbalife Nutrition ti permettono di placare la fame tra un pasto e l'altro in modo sano e
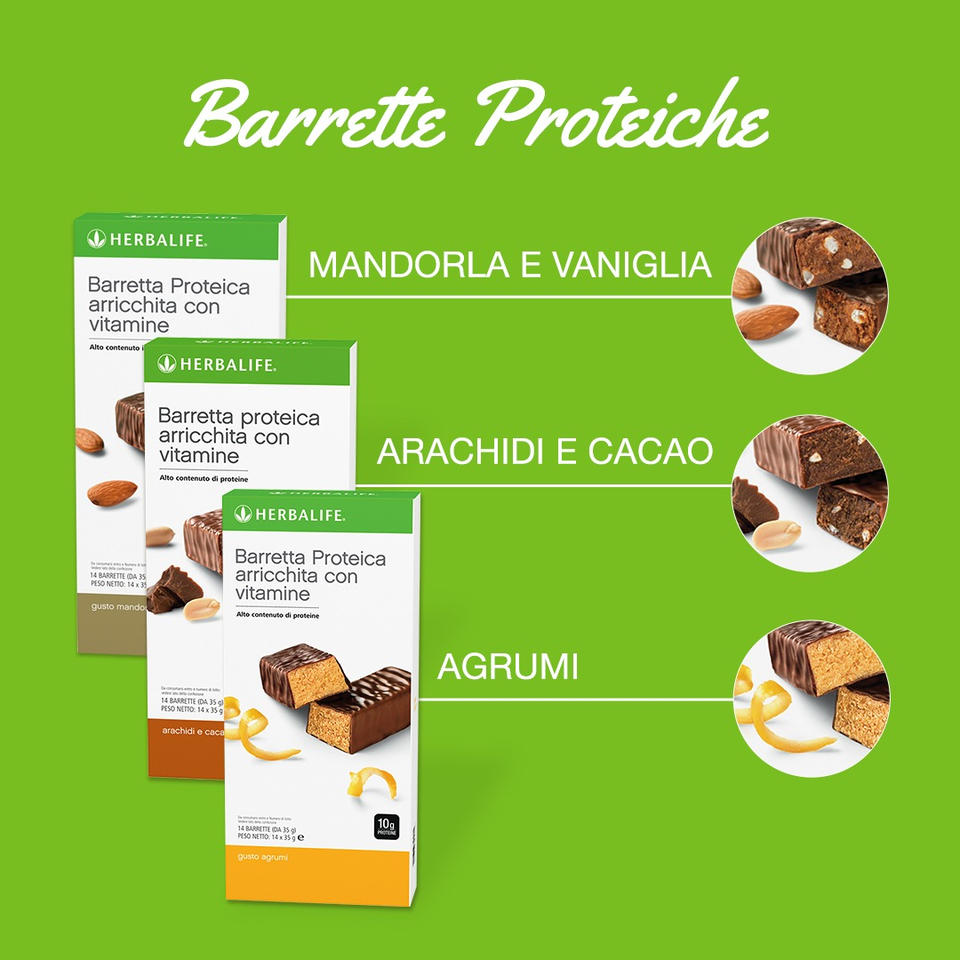
massimiliano on X: "https://t.co/0TVK2DNwk4 Le Barrette Proteiche Herbalife Nutrition sono un delizioso snack ad alto contenuto di Proteine* e Vitamine del gruppo B**. Con solo 139 calorie a barretta***. 🍫 Non pensate
