
Carburatore Solex/Brosal H32/34 PDSI 2 sinistro Fits: Type-3 1600 cc engines and replaces the Solex: 32 PDSIT-2 Type-4 engines and replaces the Solex: 34 PDSIT-2 32-34 PDSIT-2 » Garage Retrò Ricambi
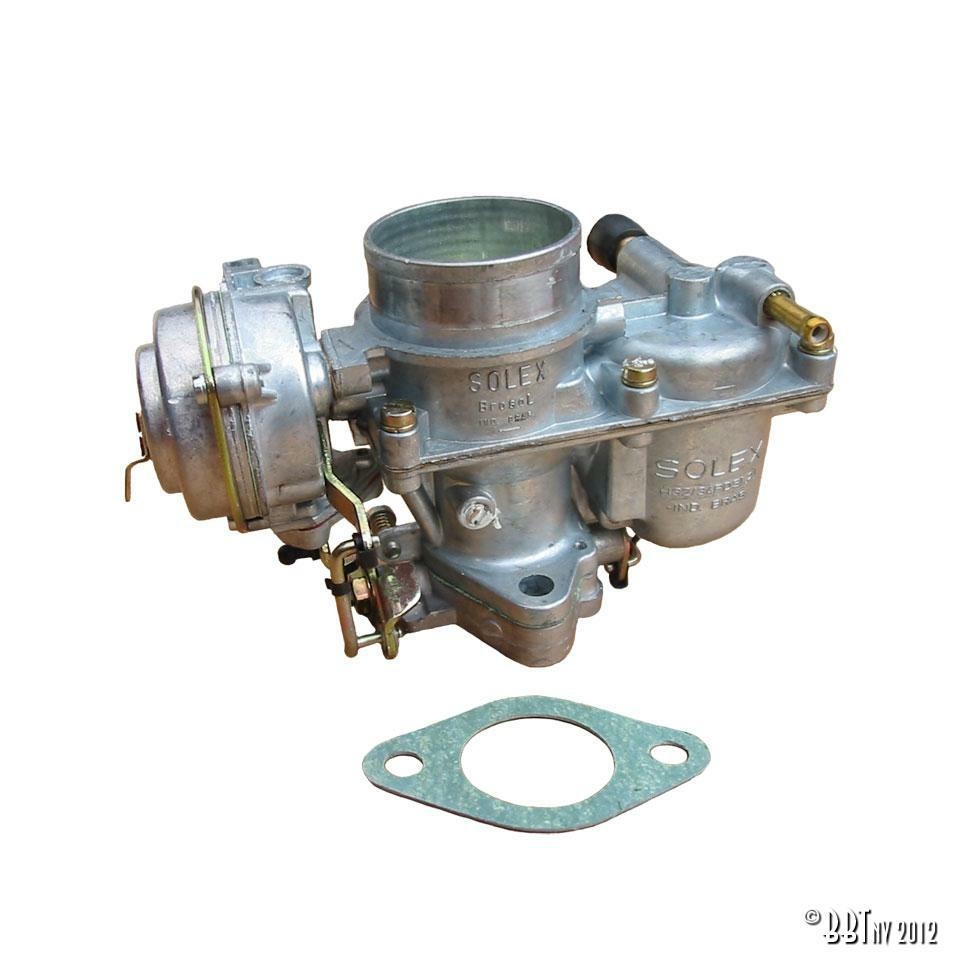
CARBURATORE SOLEX 32/34 PDSI DX T4 - Carburatori e Collettori - Dei Kafer Service - Ricambi Maggiolino, VW Classic

Carburatore Carb Carby Tipo Carburatore Carburador Solex 32 Pbic 32 Pbic Compatibile for Jeep Culatta L Motore MCS 1026 : Amazon.it: Auto e Moto
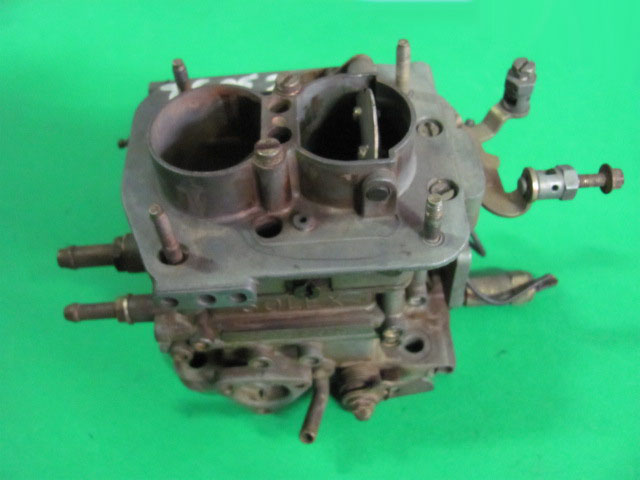
Carburatore Solex C30.32 CIC 9 usato doppio corpo Fiat 850 Special, Coupé, Spider, Panda 30 – Spitline.com
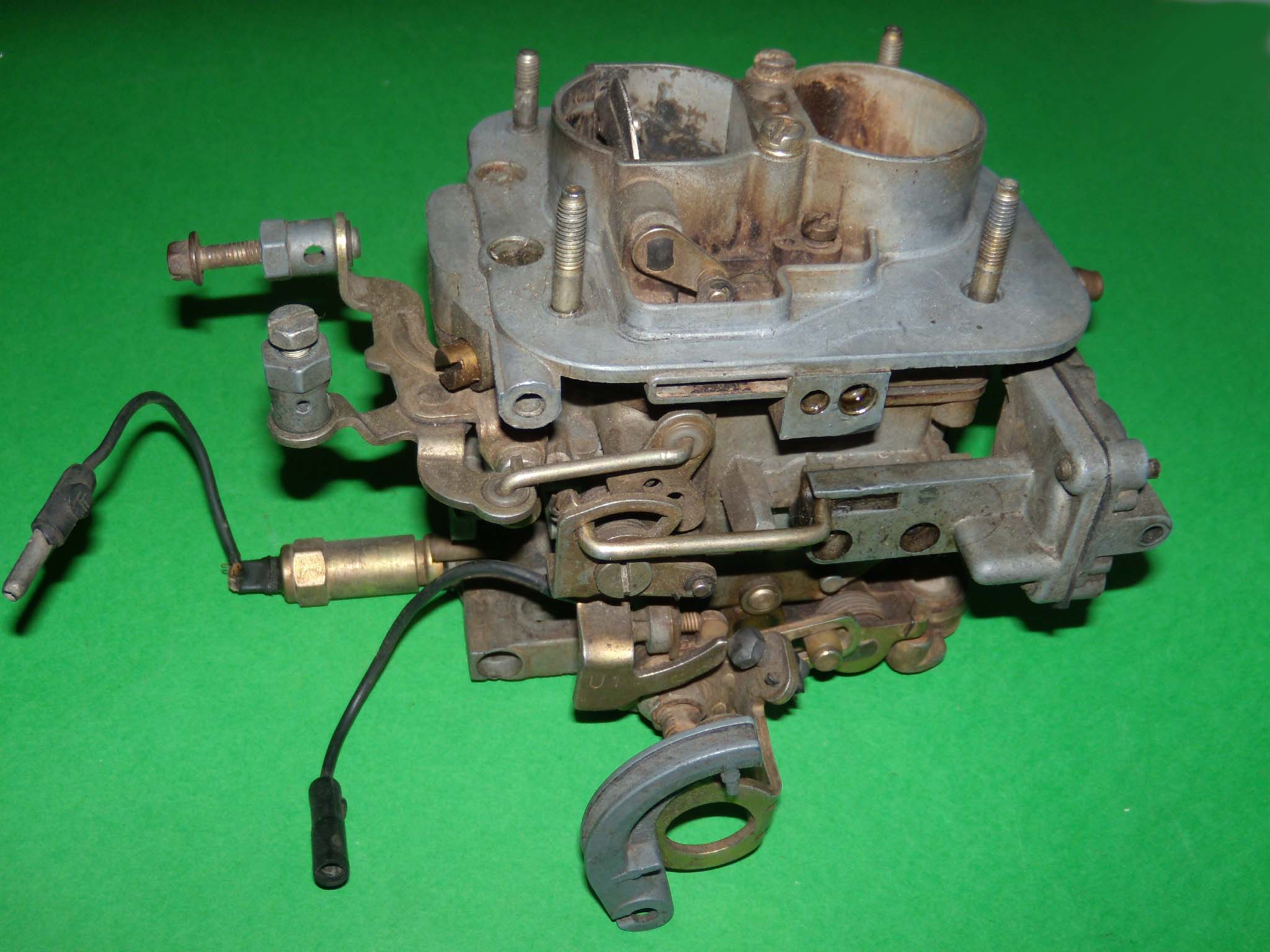