
1 Copertone 120/70-14 61 H RINF.VEE RUBBER PNEUMATICO T-MAX TMAX 500 - LIBERTY : Amazon.it: Auto e Moto

MF3294 - Copertone Anteriore Pirelli Diablo Rosso 120/70-R15 per Yamaha 500 530 560 TMAX 2008 - 2023
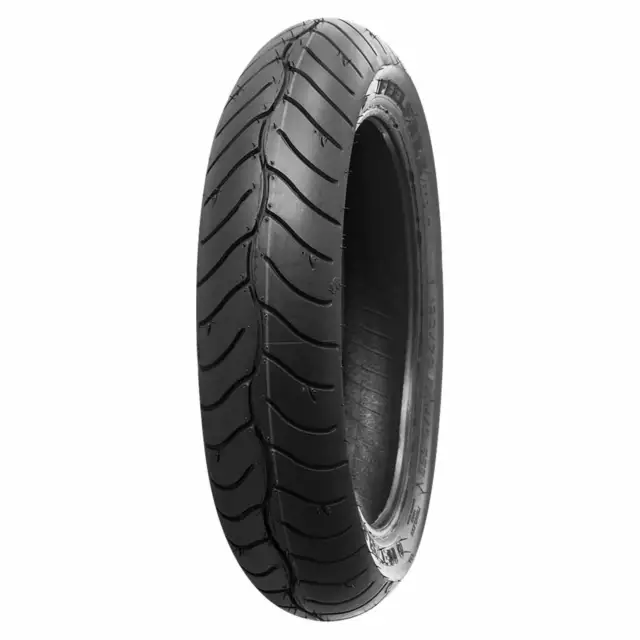
MF3296 - GOMMA Metzeler Feelfree Anteriore Yamaha 500 530 560 TMAX 2008 / 2023 EUR 94,99 - PicClick IT

Coppia gomme Scooter Pirelli Diablo rosso 120/70/15 + 160/60/15 YAMAHA T MAX 530 8019227276886 | eBay
