
Navaris Mini-Asciugamani in bambù 25x25cm - Set Bianco 6X Asciugamano Neonato e Cura Viso Bambini - Salviette Tessuto Oeko-Tex - Lavabili 30°C : Amazon.it: Prima infanzia
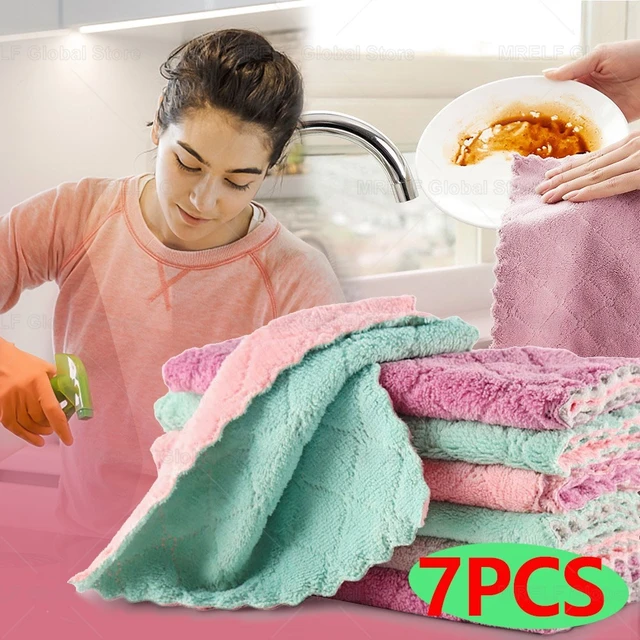
Panno in microfibra salviette magiche per la pulizia dei piatti cucina riutilizzabile cuscinetti magici addensati per

SweetNeedle Set di asciugamani - 2 asciugamani da bagno, 2 asciugamani e 2 salviette, uso quotidiano 500 g/m² filato ad anello, altamente assorbente per bagno, verde salvia (confezione da 6) : Amazon.it: Casa e cucina
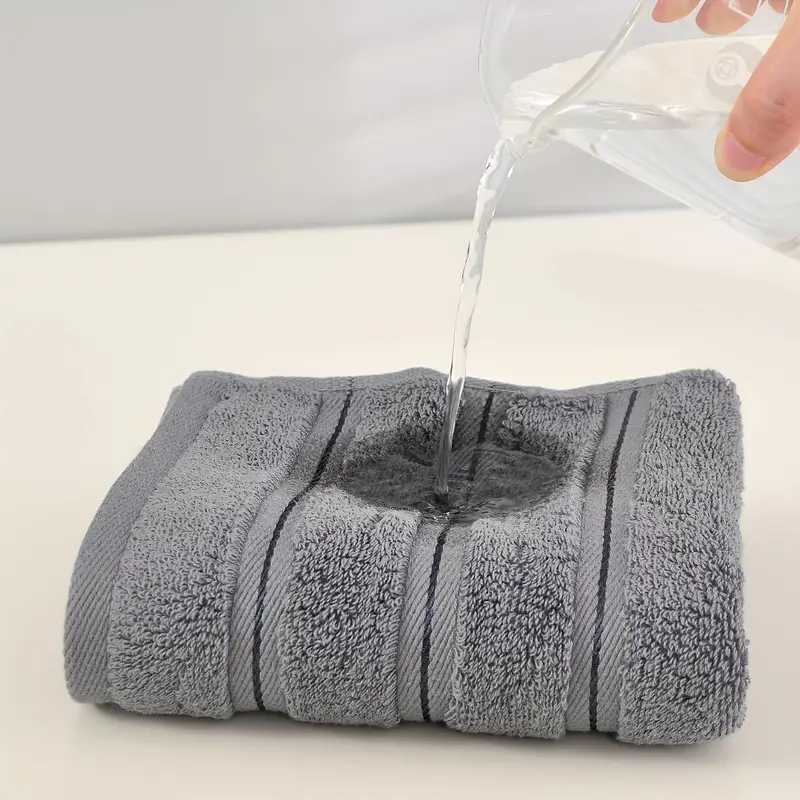
Set Di 6 Asciugamani, 2 Asciugamani Da Bagno, 2 Asciugamani Per Le Mani, 2 Salviette, Asciugamani In Puro Cotone Per Il Bagno, Accessori Per Il Bagno | Controlla Subito Le Offerte Di Oggi | Temu Italy

AkitaINK 900 pz Salviette Asciugamani Monouso di carta piegati a Z per Dispenser 6 Confezioni da 150 pz : Amazon.it: Commercio, Industria e Scienza

Liflicon 50 Salviette Asciugamani in Cotone Monouso per Viso e Corpo, Salviettine Asciutte, Salviette Pulizia Casa e Cucina, 100% Naturali, 20x20 cm : Amazon.it: Casa e cucina
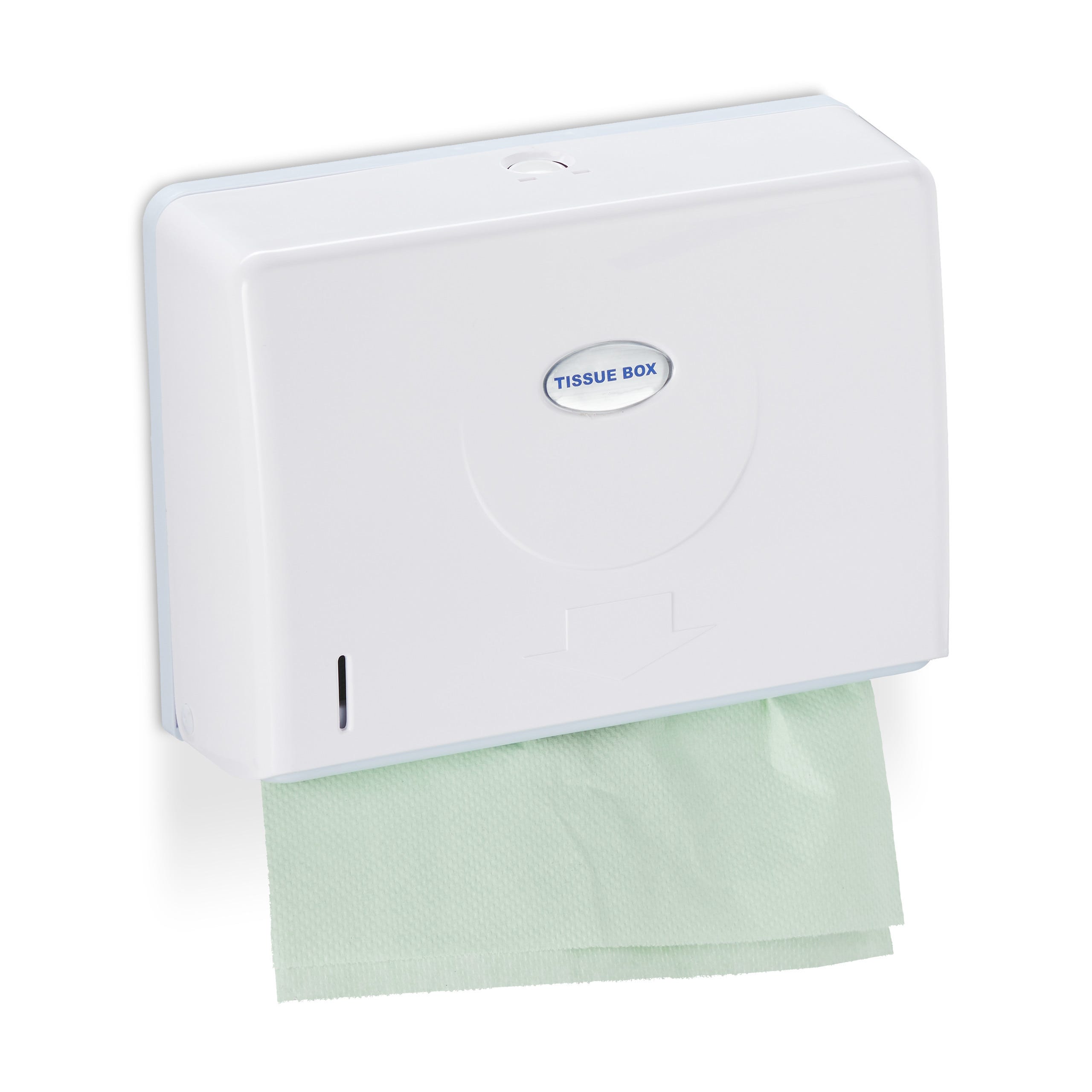
Relaxdays Distributore Carta Asciugamani, da Muro, Sistema H2, Dispenser salviette HxLxP: 20,5 x27,5 x 10 cm ca. bianco | Leroy Merlin
