
Calvin Klein CK All Eau de Toilette (unisex) 200 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.
088300104437 - Unisex - corpoecapelli - Calvin Klein Ck Be Edt Profumo Uomo Eau De Toilette Spray 200ml

Calvin Klein CK One Eau de Toilette (unisex) 200 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.

Calvin Klein CK be Eau de Toilette (unisex) 200 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.
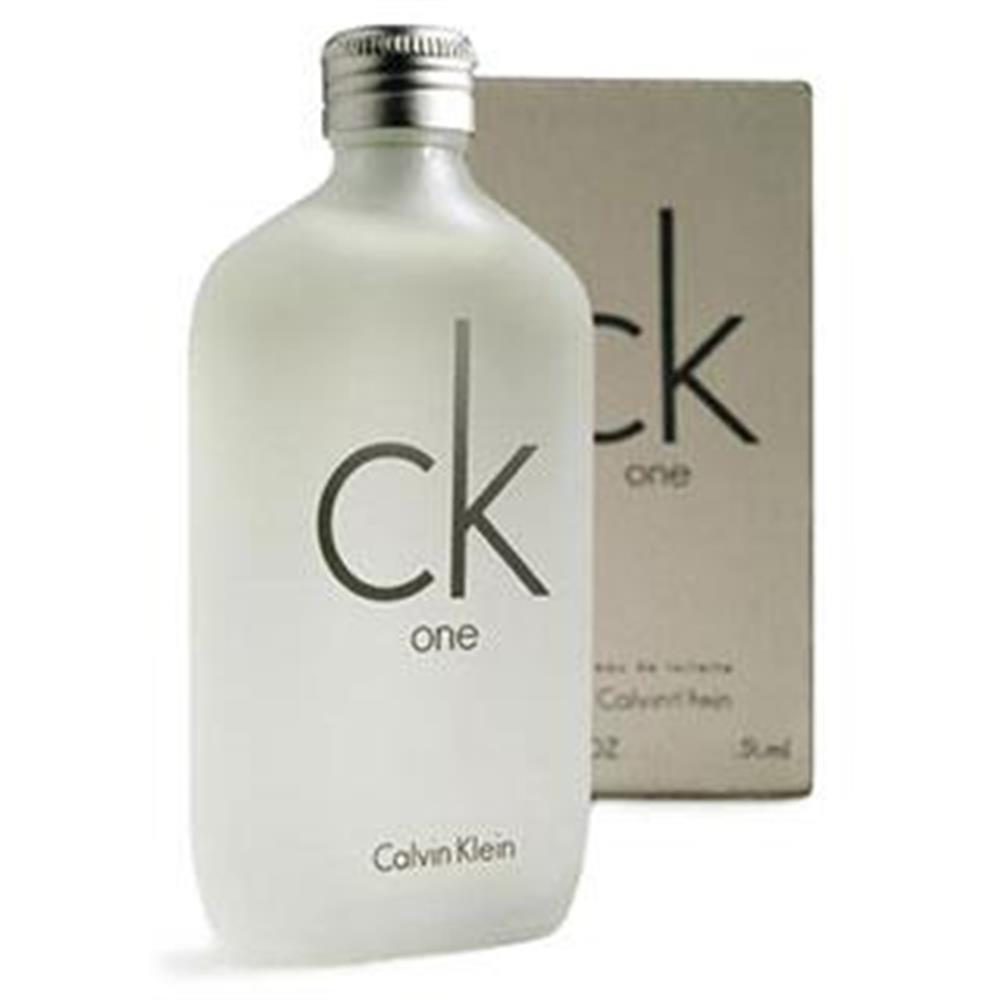
CALVIN KLEIN CK ONE 200 ml confezione originale product - Vendita tester profumi originali a prezzi super scontati. Il negozio online con il più grande assortimento di profumi. Consegna entro 48h

Calvin Klein CK One Shock For Him Eau de Toilette (uomo) 200 ml - Casa del Profumo - Profumeria premium con fragranze esclusive e cosmetici di lusso a prezzi vantaggiosi.
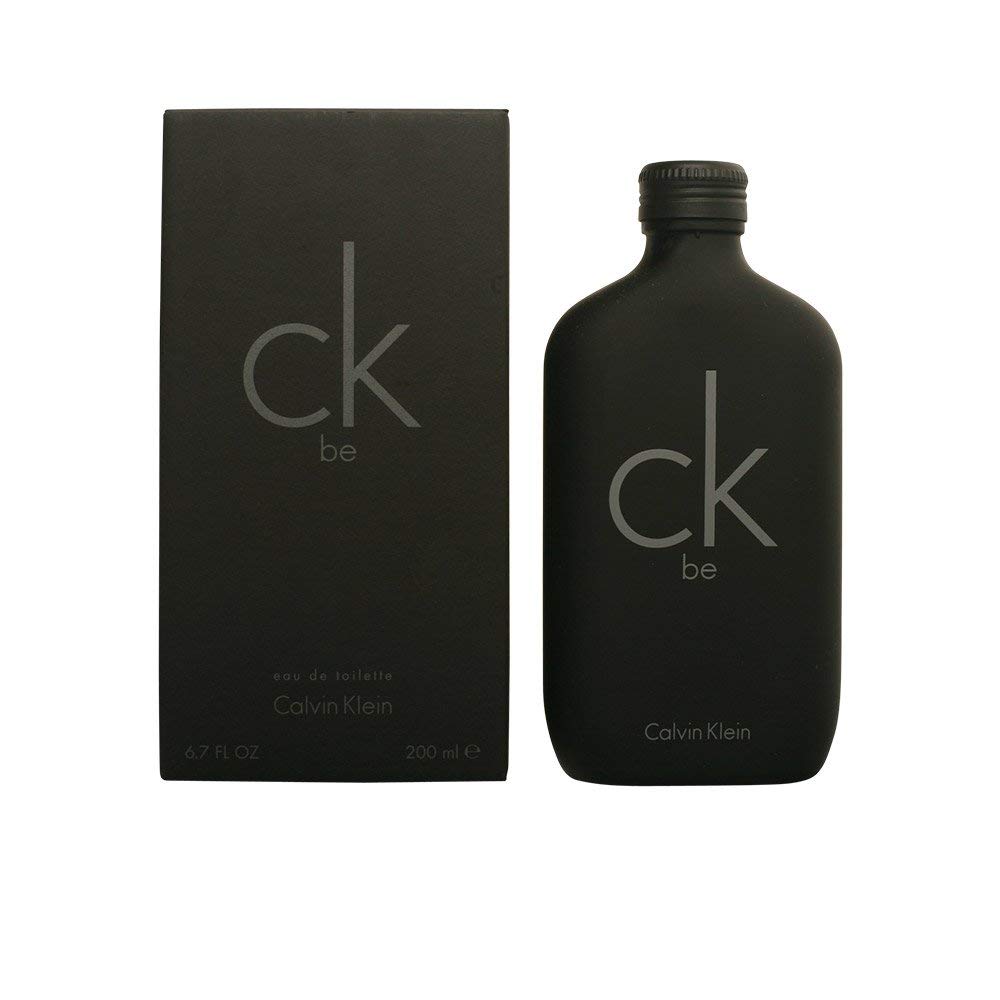